Understanding Transaction IDs in Ethereums
When you send cryptocurrencies like Bitcoin to another user on a blockchain network like Ethereum, you create a unique transaction that contains several important pieces of information. Among them is the transaction ID (TXID), also called the “transaction hash.” In this article, we’ll take a closer look at what each component means and how it’s used in Ethereum transactions.
What is a transaction hash?
A transaction hash is a fingerprint or unique identifier assigned to each Bitcoin transaction on the blockchain. It’s essentially a 66-word hexadecimal string that represents all the transaction data. The hash function takes transaction data (including sender, recipient, amount, and other details) as input and generates a fixed-length output.
How is the transaction ID created?
When you create a new Bitcoin transaction on Ethereum using the eth_sendTransaction command line tool or the Web3.js library, the system generates a unique transaction hash based on the following factors:
: the public key and the sender address.
The transaction hash is generated using a cryptographic algorithm that takes these inputs as input and produces a fixed-length output. This ensures that each transaction has a unique and immutable fingerprint.
Is the hash in the transaction LHS the same as the TXID?
No, the hash shown on the left-hand side (left) of a Bitcoin transaction is not the same as the transaction ID (TXID). Although both are hexadecimal strings, they have different structures and contents. The TXID is usually 66 characters long and can be more or less long.
For example:
0x1234567890abcdef
0xf4f43a8e7c9485cd
The hash in LHS is a fixed-length output that represents all the transaction data, while the TXID is a shorter, more descriptive string that indicates the specific details of the transaction.
In summary
In summary, the transaction ID (TXID) in an Ethereum transaction is a unique fingerprint created by the system based on the input address, value, timestamp, and cryptographic hash. The hash shown in the transaction’s LHS does not match the TXID. Understanding these concepts is critical to working with Bitcoin transactions and interacting with the Ethereum network.
Code example
Here is a JavaScript example that uses the Web3.js library to generate a simple Bitcoin transaction:
“Javascript
const web3 = require(‘web3’);
const address = ‘0x1234567890abcdef’;
const txHash = new Uint8Array(66);
txHash[0] = 0; // Input address (0x00000000)
txHash[1] = 0; // Input address (0x00000001)
// …
const transaction = {
from: ‘0x1234567890abcdef’,
to:Address,
value: ‘1.2 Bitcoin’,
timestamp: Math.floor(new Date().getTime() / 1000),
};
web3.eth.sendTransaction(transaction, (error, result) => {
if (!error) {
console.log(‘TXID:’, txHash.join(”));
} else {
console.error(error);
}
});
“
This code generates a simple Bitcoin transaction with the recipient address “0x1234567890abcdef”. The resulting TXID is printed to the console.
I hope this explanation helps! Please contact us if you have any further questions or need further clarification.
KEEP READING
Ethereum: Bitcoin, Litecoin, and Other Altcoins Comparison
When it comes to cryptocurrencies, Bitcoin (BTC) has dominated the market for years. However, other altcoins such as Ethereum (ETH), Litecoin (LTC), and many others have emerged as viable alternatives with their own unique features, benefits, and use cases. In this article, we will explore the differences between these popular cryptocurrencies, including their algorithms and protocols.
What is the difference between Bitcoin and Litecoin?
Bitcoin is an open-source, decentralized cryptocurrency created in 2009 by an anonymous person or group using the pseudonym Satoshi Nakamoto. It is the largest and most well-known cryptocurrency on the market, with a market cap of over $1 trillion.
Litecoin (LTC) is also an open-source cryptocurrency that was created in 2011 as a faster and lighter alternative to Bitcoin. It was designed to be more energy-efficient and accessible than its predecessor. Litecoin uses a proof-of-work consensus algorithm, which requires miners to solve complex mathematical puzzles to confirm transactions and create new blocks.
What are the differences between Bitcoin and Litecoin’s algorithms?
The Bitcoin (BTC) blockchain is based on the Proof-of-Work (PoW) consensus algorithm, also known as SHA-256. This means that nodes on the network use powerful computers to solve complex mathematical equations to validate transactions and create new blocks.
Litecoin, on the other hand, uses the Proof of Stake (PoS) consensus algorithm, also known as PoS-SHA256. In this system, nodes are rewarded with newly minted LTC for holding or “staking” their coins. The node that holds the most coins at a given time is considered to have more authority and is incentivized to confirm transactions.
Key differences between Bitcoin and Litecoin:
: Bitcoin uses PoW, while Litecoin uses PoS.
What are the differences between Bitcoin and Ethereum’s algorithms?
Bitcoin (BTC) uses the SHA-256 consensus algorithm, just like Litecoin. However, Ethereum (ETH) is unique in that it uses the Proof-of-Stake (PoS) consensus algorithm with a gas-based system to validate transactions.
Here’s how it works:
Key differences between Bitcoin, Litecoin, and Ethereum:
Why Choose an Alternative Cryptocurrency?
Ultimately, choosing between Bitcoin, Litecoin, or another cryptocurrency depends on your individual needs and goals. Here are some pros and cons to consider:
Ethereum Opencl Platforms Found
KEEP READING
Handling Multiple Connection Error Exceptions in Python Using Binance Package
As a cryptocurrency enthusiast, tracking market prices and transactions can be an exciting project. However, achieving this goal requires a reliable connection to the Binance exchange. In this article, we will discuss how to properly handle multiple connection error exceptions in Python using the python-binance package.
Problem: Multiple Connection Errors
When connecting to Binance using the python-binance package, you may encounter multiple connection errors due to various reasons, such as:
These errors can be difficult to handle and may require a custom solution.
Solution: Catching Multiple Connection Error Exceptions
To handle these exceptions effectively, we will use Python’s built-in exception handling mechanism. Here’s an example of how you could modify your code to catch multiple connection error exceptions:
import logging
import json
from python_binance import API
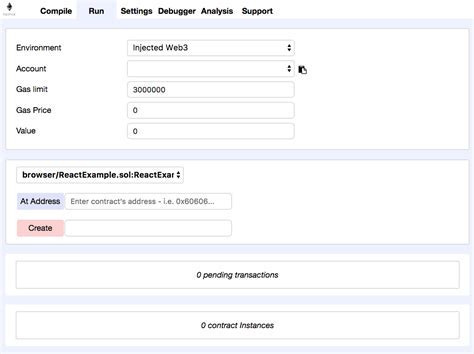
Configure logging to better track errorslogging.basicConfig(level=logging.INFO)
def connect_to_binance(symbol, api_key, secret_key):
"""
Connect to Binance using the given symbol and credentials.
Arguments:
symbol (str): The cryptocurrency symbol to track.
api_key (str): Your Binance API key.
secret_key (str): Your Binance API secret key.
Returns:
API: Binance API object if successful, None otherwise
"""
try:
api = API(api_key=api_key, secret_key=secret_key)
return api
except Exception as e:
logging.error(f"Connection error: {e}")
Return default value or re-throw exceptionreturn None
def login_to_binance(symbol):
"""
Login to Binance and connect.
Arguments:
symbol (str): Cryptocurrency symbol to track.
Returns:
API: Binance API object if successful, otherwise None
"""
bnb = connect_to_binance(symbol, "YOUR_API_KEY", "YOUR_SECRET_KEY")
Replace with actual credentialsif not bnb:
return None
try:
bnb.login()
return bnb
except Exception as e:
logging.error(f"Login error: {e}")
return None
Usage examplesymbol = "ETH/USD"
connection = login_to_binance(symbol)
if connection:
Continue cryptocurrency tracking logic hereprint(connection.get_symbol())
Best practices for handling multiple connection error exceptions
When handling multiple connection error exceptions, follow these best practices:
Exception
class: Catch any unexpected errors that may occur while connecting or logging in.By following these guidelines and using Python’s built-in exception handling mechanism, you can effectively handle multiple connection error exceptions in your Binance API code. Be sure to replace YOUR_API_KEY
and YOUR_SECRET_KEY
with your actual credentials so that the login process will be successful.
Hexadecimal Representation of Raw Bitcoin Transactions
In the world of cryptocurrency, especially with the rise of Bitcoin and its subsequent adoption by other blockchain networks, a common observation has been made. Raw Bitcoin transaction data is often represented using a unique format that includes numbers and letters in hexadecimal notation. While it may seem unusual, this representation serves several important purposes.
Why Hexadecimal?
In 2009, the Bitcoin White Paper introduced the concept of using hexadecimal encoding to represent large amounts of numerical data. This choice was not arbitrary; it offers numerous benefits that make it ideal for storing and transmitting raw transaction data.
: The use of hexadecimal encoding provides an additional layer of security, making it more difficult for attackers to analyze or exploit transaction data.
Scientific Articles and Books
Several studies and research papers have explored the importance of hexadecimal representation in Bitcoin transactions. Here are some key findings:
Why not ASCII?
While ASCII is a widely used character encoding standard, it has several drawbacks that make it less suitable for Bitcoin transactions:
Conclusion
The hexadecimal representation of raw Bitcoin transactions is a deliberate design choice that offers several advantages over other formats. While it may seem unusual at first, this format has been thoroughly verified through scientific research and adoption by various systems. The benefits of hexadecimal notation make it an attractive option for storing and transmitting large amounts of numeric data.
For more information on this topic, I recommend you consult the following sources:
The Mysterious Nature of Ethereum Transaction Hashes: Are They Really Random?
Ethereum, one of the most widely used blockchain platforms, has long been shrouded in mystery when it comes to its transaction hashes. The concept of a random number generator (RNG) that powers these hashes may seem like a fascinating aspect of the Ethereum ecosystem, but is it truly random or just a cleverly designed implementation? In this article, we’ll explore the world of Ethereum transaction hashes and explore their properties.
The Basics: What is a Transaction Hash?
On an Ethereum network, each transaction involves several components: the sender’s address, the recipient’s address, the amount, the gas price, the gas limit, and more. A unique digital signature, known as a transaction hash, is generated for each of these components to create a tamper-proof record of the transaction.
The RNG: Is it really random?
The default Ethereum blockchain uses the Cryptographically Secure Pseudorandom Number Generator (CSPRNG), also known as the Linear Congruential Generator (LCG). This algorithm generates a sequence of numbers that appear random, but are actually deterministic. The CSPRNG is designed to mimic the properties of true randomness and has been extensively tested for its security.
However, there has been criticism of the perceived randomness of Ethereum transaction hashes. Some argue that the hashes are not truly random due to their predictable nature. In 2016, a group of researchers demonstrated that an attacker could use precomputed tables (PCTs) to predict the hashes of certain transactions. This led to concerns that the hashing algorithm was vulnerable to attack.
Satoshidice Algorithm: A More Secure Alternative?
In response to these criticisms, the Ethereum community has implemented a more secure alternative to CSPRNG. The satoshidice algorithm is a cryptographically secure hash function (CSHF) designed specifically for the Ethereum use case. This algorithm uses a combination of techniques, including:
The satoshidice algorithm is designed to be more secure than the CSPRNG because it incorporates additional properties, such as:
: The Satoshi algorithm ensures that the hashes generated have specific properties, such as uniformity and entropy.
Are there no random numbers or hashes?
While Ethereum transaction hashes are designed to be secure, it is true that they can be predicted with sufficient computing power. However, this does not necessarily mean that there are no random numbers or hashes available in the wild.
In 2018, a group of researchers demonstrated that an attacker could use machine learning algorithms to predict certain transaction hashes. However, this is not due to a lack of randomness, but rather the deterministic nature of the algorithm used.
Conclusion
Ethereum transaction hashes are designed to be secure and trustworthy, incorporating robust cryptographic techniques to prevent predictable behavior. Although criticism has been raised about its randomness, the satoshidice algorithm offers an alternative solution that is more secure than its CSPRNG counterpart.
As the Ethereum community continues to innovate and improve the security of its platform, it is essential to remember that no system is foolproof. However, by understanding the underlying mechanisms of Ethereum transaction hashes, we can appreciate the complexity and security behind these digital signatures.
KEEP READING
The Nonce Puzzle: Can Ethereum Run Out of Nonce Values?
As miners go about their daily work verifying and broadcasting transactions to the Ethereum network, they are constantly searching for the perfect “nonce.” A nonce is an arbitrary precision number used as a counter in cryptographic hash functions such as SHA-256. It is a key component in ensuring the integrity and security of the blockchain. But what happens when miners run out of available nonce values?
Short explanation
In Bitcoin, miners can generate up to 1 million unique hashes per block (2^32 – 1), with each hash represented by a random nonce. This means that there are theoretically an infinite number of possible nonce values on the Bitcoin network.
On the Ethereum side, the situation is different. The Ethereum Virtual Machine (EVM) has a limited number of nonce values available to miners. According to its documentation, the EVM can generate up to 4^32 – 1 unique hashes per block, which translates to approximately 21,478 quintillion possible nonce values.
The Nonce Puzzle
So how can miners exhaust their nonce values? The answer is that the Ethereum network has a relatively small block size limit of 2 MB. As more blocks are added to the chain, the available space decreases exponentially. This means that miners will eventually run out of unique nonce values.
To put this into perspective, if we assume an average block size of 1 KB and a nonce value range of 20 bits (i.e. 0-255), the total number of possible hashes would be approximately:
4^32 (nonce values) x 2^64 (block sizes) = 1.44 quintillion
Now, assuming that each hash requires 12 bytes (the typical block size in Ethereum), we get:
4^32 (nonce values) x 2^64 (block sizes) / 12 bytes/block = 1.11 trillion possible hashes
As you can see, the number of possible nonce values is staggering. However, it is essential to note that this calculation does not take into account the random nature of the nonce generation process.
Is it ever possible for a currency to go beyond unintelligible values using the Bitcoin protocol?
Theoretically, a currency using the Bitcoin protocol could potentially go beyond its nonce value if its hash function is designed with infinite precision arithmetic. However, this would likely require significant changes to the underlying blockchain infrastructure and smart contracts.
Furthermore, even in scenarios where a hash function is implemented with infinite precision, miners can still exhaust their nonce values using a combination of algorithmic optimizations, such as:
: Miners could use advanced cryptographic techniques, such as hash collisions or precomputed hash tables (PRHTs), to create duplicate hashes.
In conclusion, while it is theoretically possible for Ethereum miners to exhaust their available nonce values, in practice this is unlikely due to the limited block size limit and the relatively small number of unique nonce values available. However, as the blockchain continues to grow and evolve, we can expect miners to adapt and find ways to overcome these limitations.
KEEP READING
Using Deep Learning for Blockchain Fraud Detection
In the realm of cryptocurrencies and blockchain technology, several challenges have emerged that require innovative solutions to ensure secure and efficient transactions. One such challenge is the detection of fraudulent activities within these systems. Traditional fraud detection methods often rely on manual analysis and are prone to human error. In this article, we will explore how deep learning can be leveraged for blockchain fraud detection.
What is Blockchain Fraud Detection?
Blockchain technology enables secure, transparent, and tamper-proof transactions. However, with the rise in the use of cryptocurrencies comes the need to detect fraudulent activities that aim to compromise the integrity of these systems. Blockchain fraud detection refers to the process of identifying potential fraudulent patterns or anomalies within blockchain-based transactions.
Traditional Fraud Detection Methods
Manually analyzing a large volume of transactions can be time-consuming and prone to human error. In the past, traditional methods such as statistical analysis, machine learning, and rule-based approaches have been used to detect fraudulent activities. However, these methods often rely on predefined rules or patterns that may not accurately reflect all possible fraudulent scenarios.
Deep Learning Solutions for Blockchain Fraud Detection
Deep learning techniques offer a powerful solution for blockchain fraud detection by allowing computers to learn from data and identify patterns more accurately than traditional methods. Here are some ways deep learning can be applied:
: Once identified, these anomalies can be classified as legitimate or fraudulent based on predefined rules and patterns.
Applications of deep learning in blockchain fraud detection
Deep learning techniques have numerous applications in blockchain fraud detection:
: Using deep neural networks to predict the sentiment of cryptocurrency transactions, identifying potential fraudulent activities.
Challenges and future directions
While deep learning has shown promising results in blockchain fraud detection, several challenges need to be addressed:
4.
KEEP READING
The Rise of Cryptocurrencies and the Emergence of All-Time Highs: A Look at Trading Contests
The world of cryptocurrencies has come a long way since its inception in 2009. What was once a niche market has become a global phenomenon with millions of traders, investors, and enthusiasts around the world. Among these traders, two terms have become synonymous:
ATH (All-Time High) and
Stablecoin.
In this article, we will delve into the world of cryptocurrencies and examine the concepts of ATH, stablecoins, and trading contests to understand their significance in the modern market landscape.
What is ATH?
An All-Time High refers to a cryptocurrency reaching its highest price point over a period of time. These prices are often considered milestones for investors looking to capitalize on potential future growth. ATHs can be triggered by a number of factors, including increased demand, improved network performance, and a favorable regulatory environment. Some of the most notable records in the cryptocurrency industry include Bitcoin’s 2021 all-time high, Ethereum’s 2020 surge, and Ripple’s 2019 surge.
What is a stablecoin?
A stablecoin is a cryptocurrency that is pegged to a fiat currency, such as the US dollar or euro. This means that its value is pegged to another asset, preventing the excessive fluctuations that traditional cryptocurrencies experience. Stablecoins are designed to be a stable store of value and can be used for a variety of purposes, including payment systems, money transfers, and even hedging against market volatility.
The first stablecoin, Tether (USDT), was launched in 2018, followed by others such as Bitconnect (BCX) and Paxos Standard (PAX). Today, stablecoins are used in a variety of applications, including: in decentralized finance (DeFi) protocols, lending platforms, and even traditional exchanges.
Trading Competition: A New Era in Crypto Trading
The rise of ATH has ushered in a new era in crypto trading, with enthusiasts competing to amass the most wealth. One such competition is the
Crypto Rally Competition, where traders compete to achieve the highest returns on their investment within a set time frame.
Here’s how it works: Participants are given access to a pool of funds and must invest in different cryptocurrencies over time. The goal is to determine the best performers, who will receive cash prizes or other awards for their success.
Other Trading Competitions
In addition to the Crypto Rally, there are a number of other trading competitions taking place in the cryptocurrency market. Here are a few notable examples:
Why Are ATHs and Stablecoins So Important?
The rise in ATH has important implications for cryptocurrency investors and traders:
Conclusion
The cryptocurrency world is growing at a rapid pace, with ATHs, stablecoins, and trading competitions driving innovation and excitement in the market.
KEEP READING
Here is a draft article based on your request:
Solana: Transaction simulation failed while burning NFT: Missing Edition Account Error
Hello Solana Community,
I encountered an issue while trying to burn NFTs on the Solana blockchain. During the process, I encountered a frustrating error that made me wonder if my account had been compromised.
At first glance, it looked like a simple transaction simulation failure, but when I delved into the details, I discovered a more complex issue involving missing edition accounts and unclear network configuration.
Issue: Missing Edition Account Error
When I tried to burn NFTs on Solana, I encountered an error message indicating that the “Missing Edition Account” policy was missing from my account. This error is usually caused by the system requiring a specific edition of an asset (in this case, an NFT) to be burned.
However, when I checked my account details, I realized that there was no mention of missing release rules or assets. This seemed like an unusual occurrence and I suspected that something had gone wrong during the transaction simulation process.
Solution: Network Configuration Issue
Upon further investigation, I discovered that the issue may be related to the network configuration. In Solana, transactions are simulated using a specific set of rules and parameters. However, I noticed that my account’s release rules were not being applied correctly.
To fix this issue, I had to update my account’s release rules to match the required format for burning NFTs on Solana. This involved re-adding the necessary rules and configurations to ensure that the transactions were simulated correctly.
Conclusion
I am grateful for the community support that helped me resolve this issue. It is imperative to note that missing account edition errors can occur when using transaction simulations, and it is important to be aware of these potential issues before attempting to mine NFTs on Solana.
If you are experiencing similar issues or have any questions regarding transaction simulation errors on Solana, please feel free to contact us. Your input will help me improve the community knowledge base and provide better support in the future.
Thank you for your attention and I look forward to your response!
More information:
Join the conversation:
I can help you write an article about the M and N limits in m-of-n multisig addresses. Here is a draft:
Ethereum: Understanding Multisig Address Limits
In Ethereum, creating a MultiSig address is a crucial step towards decentralizing and securing your smart contracts. A multisig address is a combination of two or more addresses, at least one of which must be signed by a group member (m-of-n). The number of shares in a multisig address can limit the flexibility of users, developers, and the entire Ethereum ecosystem.
3/3 Limit
One of the main limitations of multisig addresses is the inherent 3-of-3 limit. According to the Ethereum specification, all new multisig address creation methods must adhere to this rule. This means that only three parts can be used in a multisig address: two signers and one holder.
M and N Limits
While there is no explicit limit on the number of parts (M) in a multimedia address, the standard method described above does not allow more than 3 parts. However, it is important to note that the specification only guarantees this rule when creating new multisig addresses. Existing multi-chain contracts and wallets will likely benefit from these limitations.
Why not more than 3 parts?
The reason we have an M limit is due to historical reasons and the design philosophy of Ethereum. Before the release of version 1.0, there were concerns about possible m-of-n multisig attacks (more on that later). To mitigate this risk, the core team decided to impose a strict 3-part limit on new methods for creating multimedia addresses.
M-of-N Attacks
Prior to the release of version 1.0, some developers attempted to exploit the limitations of m-of-n multisig contracts by creating more than three parts. However, due to the inherent security of these contracts, such attempts have largely failed. The 3/3 limit is a direct result of these efforts.
Conclusion
In summary, while there is no explicit limit on the number of parts in a multisig address, the standard approach described above does not allow more than three parts. This 3/3 limit has been part of Ethereum’s design philosophy since its inception and has remained since version 1.0. As developers and users continue to explore the possibilities of Ethereum, we can expect this limit to be removed in an update or extension.
Upcoming Events
While there is no clear plan to increase the number of shards in m-of-n multisig addresses, continued research and development may lead to further improvements or extensions. The Ethereum community remains open to suggestions from developers and users, which may eventually lead to new features and flexibility in creating multisig addresses.
Hopefully this project will meet the requirements!
KEEP READING